Firebase is a powerful backend-as-a-service (BaaS) platform created by Google. It provides a wide range of tools for the easy development, management, and growth of web and mobile apps. Known for its real-time data handling and extensive features including analytics, crash reporting, and machine learning, Firebase significantly speeds up development processes and efficiently manages application data. Paired with React Native, Firebase becomes an invaluable asset for developers attempting to create efficient cross-platform applications.
with Expert Firebase
& React Development. With over a decade of experience,
Talentelgia excels in delivering
cutting-edge development services.
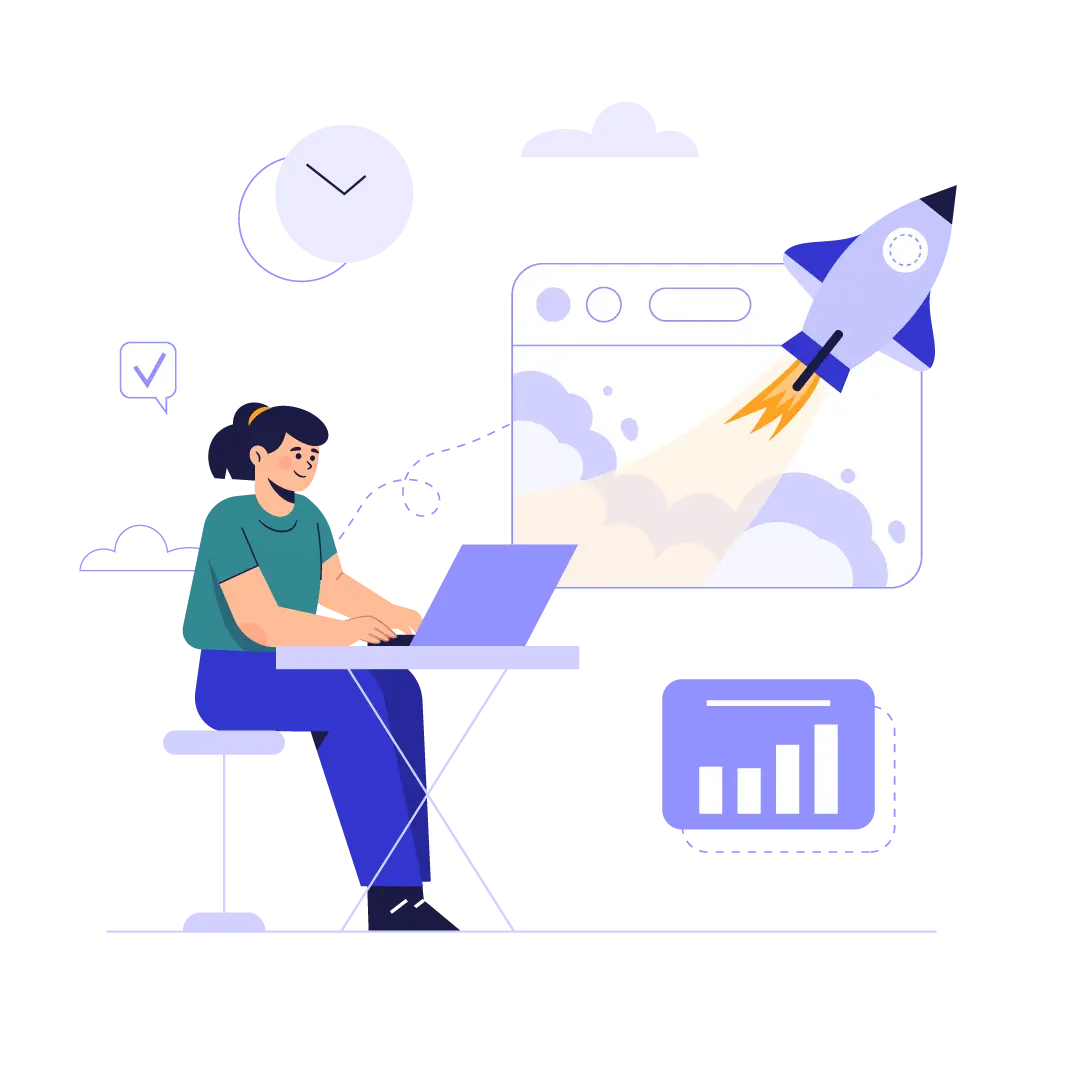
This tutorial provides an in-depth blog on integrating Firebase within a React Native app.
Benefits of Using Firebase with React Native
Integrating Firebase with React Native brings forth a multitude of benefits that significantly bolster both app development and operational performance. Below is a thorough look into the advantages:
Real-time Data Synchronization
Firebase is renowned for its real-time database capability which promotes uninterrupted data flow across all platforms and devices. Every alteration within the database is sent instantaneously to all linked clients. This feature is indispensable for apps that rely on real-time information updates such as chat applications or live event tracking. The instantaneous data reflection not only cultivates an enhanced user experience by making the latest information readily available but also maintains uniformity of data across all devices, eliminating the need for manual updates.
Authentication
The robust, secure authentication infrastructure provided by Firebase drastically eases the management of user identities. By supporting a multitude of authentication methods including social logins (Google, Facebook, Twitter) and traditional e-mail sign-ups, Firebase simplifies complex authentication operations. Designed for both robustness and ease of implementation, Firebase Authentication handles routine tasks like user management, password resets, and email confirmations efficiently, freeing developers from potential security concerns and complex setup procedures.
Cloud Functions
Firebase Cloud Functions serve as a nimble backend solution where you can execute code in response to events initiated by Firebase services or HTTP requests. This serverless computing enables automated responses to changes in Auth, Firestore, or the Realtime Database, facilitating tasks such as notifications, data updates, or external integrations without managing a private server. This capacity allows developers to concentrate more on the front end while Firebase smoothly operates the backend operations.
Analytics and Monitoring
With Firebase, developers gain access to detailed analytics and monitoring tools that are crucial for tracking user engagement and app responsiveness. Firebase Analytics delves into user interaction patterns, providing essential insights that help in refining app functionalities and interfaces. Additionally, Firebase can be linked with platforms like Google Ads to improve marketing by allowing for precise targeting of users. Firebase Performance Monitoring helps identify and remedy app performance issues, ensuring the app operates smoothly without any delays or interruptions.
By merging these functionalities with the cross-platform adaptability offered by React Native, developers achieve heightened efficiency and reduced development timelines. React Native facilitates the maintenance of a singular codebase for both iOS and Android platforms, slashing development resources and costs. When used alongside Firebase, it amplifies productivity by streamlining backend tasks such as data sync, user authentication, and server handling.
As for scalability, Firebase automatically adjusts to an app’s growth demands, accommodating expanding user bases smoothly. This adaptive nature, combined with robust real-time databases and performance analytics, ensures that the application performs efficiently and remains proactive, thereby boosting user satisfaction and engagement.
Setting Up Your Firebase Project with React Native
Step 1: Create a New Firebase Project
- Navigate to the Firebase Console.
- Select “Add project” and proceed with the provided setup instruction steps.
Step 2: Adding Firebase to Your Android App
Premier Firebase &
React Development With over a decade of experience,
Talentelgia excels in providing
top-notch services.
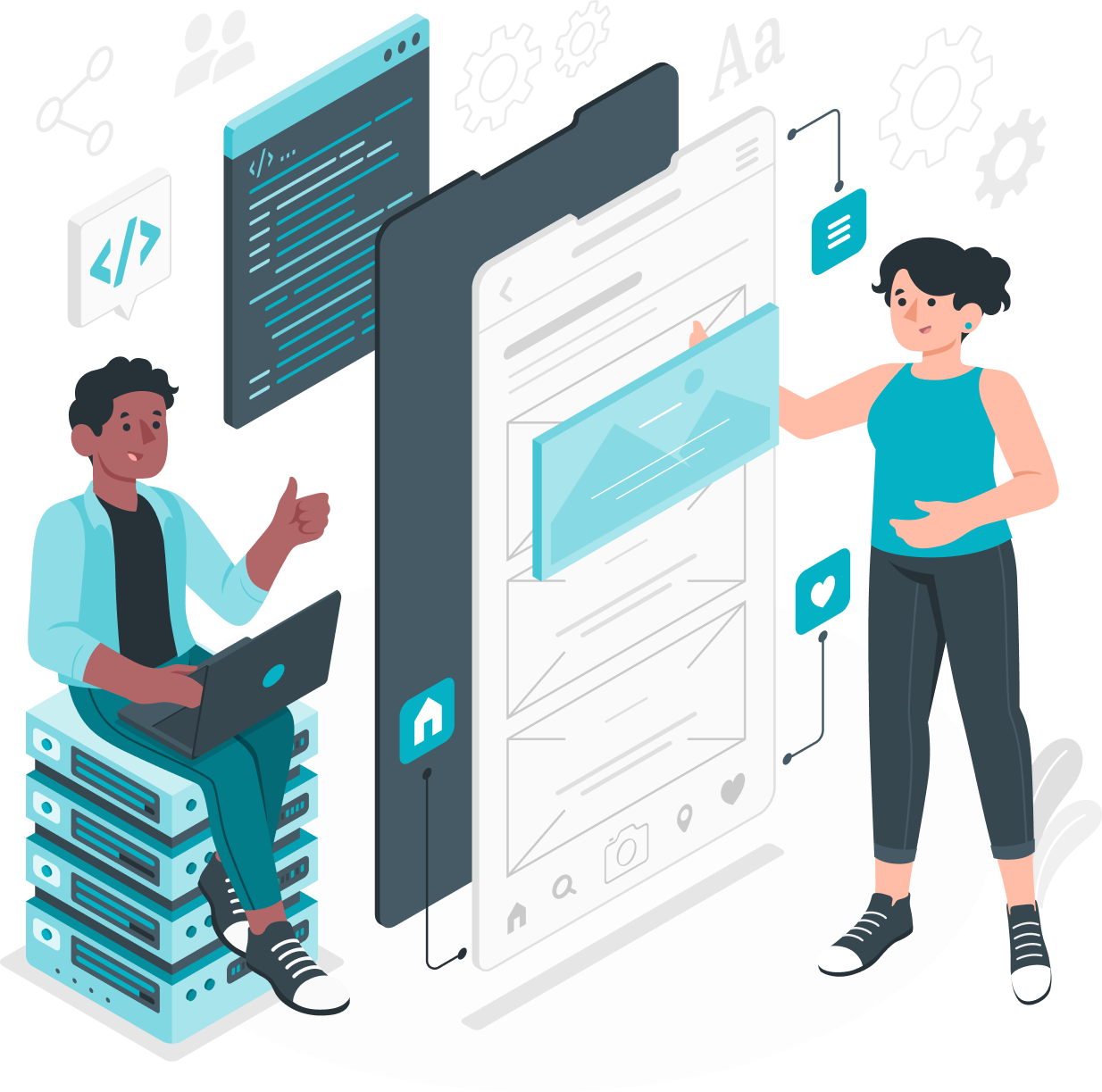
- Within your Firebase project, choose “Add app” and opt for the Android platform.
- Follow all setup steps carefully, input your app specifics, and ensure accuracy.
- Download the google-services.json file and implement it in your app’s Android/app directory.
Configuring React Native App
Step 1: Installing Dependencies for Firebase
npm install @react-native-firebase/app
npm install @react-native-firebase/auth
npm install @react-native-firebase/firestore
# Add other necessary dependencies
Step 2: Setting Up Firebase with React Native
//Initialize Firebase in your app’s entry point (usually index.js)
JavaScript
import {AppRegistry} from 'react-native';
import {App} from './App'; // Your main app component
import {name as appName} from './app.json';
import {firebase} from '@react-native-firebase/app';
firebase.initializeApp({
// Your Firebase config
});
AppRegistry.registerComponent(appName, () => App);
Integrating Firebase Authentication
Registration with Firebase Auth
JavaScript
import {firebase} from '@react-native-firebase/auth';
const registerUser = async (email, password) => {
try {
await firebase.auth().createUserWithEmailAndPassword(email, password);
// Handle success
}
catch(error){
// Handle error
}
};
Login with Firebase Auth
JavaScript
const loginUser = async (email, password) => {
try {
await firebase.auth().signInWithEmailAndPassword(email, password);
// Handle success
}
catch(error){
// Handle error
}
};
Conclusion
Using Firebase with React Native makes backend development easier and improves the performance and growth potential of apps. This blog has given you the basic knowledge needed to use Firebase in your React Native projects effectively. To get a better understanding and discover more about the additional features and tools that Firebase offers, it’s recommended to read through Firebase’s comprehensive documentation. By doing so, you can improve and fine-tune your mobile apps, making them more robust and easier to use.